Write a C program to input elements in an array and print array using pointers. How to input and display array elements using pointer in C programming.
Example
Input
Input array size: 10 Input elements: 1 2 3 4 5 6 7 8 9 10
Output
Array elements: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
Required knowledge
Basic C programming, Array, Pointers, Pointers and Array
Learn to input and print array without pointer.
How to access array using pointer
Array elements in memory are stored sequentially. For example, consider the given array and its memory representation
int arr[] = {10, 20, 30, 40, 50};
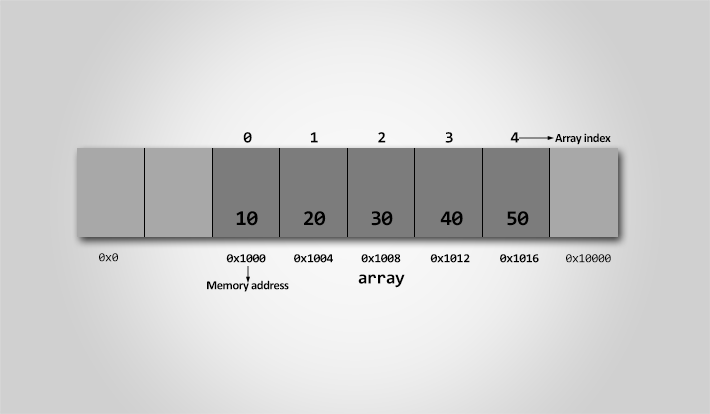
If you have a pointer say ptr
pointing at arr[0]
. Then you can easily apply pointer arithmetic to get reference of next array element. You can either use (ptr + 1)
or ptr++
to point to arr[1]
.
Program to input and print array elements using pointer
/**
* C program to input and print array elements using pointers
*/
#include <stdio.h>
#define MAX_SIZE 100 // Maximum array size
int main()
{
int arr[MAX_SIZE];
int N, i;
int * ptr = arr; // Pointer to arr[0]
printf("Enter size of array: ");
scanf("%d", &N);
printf("Enter elements in array:\n");
for (i = 0; i < N; i++)
{
scanf("%d", ptr);
// Move pointer to next array element
ptr++;
}
// Make sure that pointer again points back to first array element
ptr = arr;
printf("Array elements: ");
for (i = 0; i < N; i++)
{
// Print value pointed by the pointer
printf("%d, ", *ptr);
// Move pointer to next array element
ptr++;
}
return 0;
}
But wait before moving on to next program, there is another way to write the above program. I must say the better way to deal with arrays using pointer is, instead of incrementing pointer use pointer addition.
Program to input and print array using pointers – best approach
/**
* C program to input and print array elements using pointers
*/
#include <stdio.h>
#define MAX_SIZE 100 // Maximum array size
int main()
{
int arr[MAX_SIZE];
int N, i;
int * ptr = arr; // Pointer to arr[0]
printf("Enter size of array: ");
scanf("%d", &N);
printf("Enter elements in array:\n");
for (i = 0; i < N; i++)
{
// (ptr + i) is equivalent to &arr[i]
scanf("%d", (ptr + i));
}
printf("Array elements: ");
for (i = 0; i < N; i++)
{
// *(ptr + i) is equivalent to arr[i]
printf("%d, ", *(ptr + i));
}
return 0;
}
Note:
(ptr + i)
is equivalent to&ptr[i]
, similarly*(ptr + i)
is equivalent toptr[i]
. Also you can use(i + ptr)
,i[ptr]
all means the same. Read more about array indexes and pointer.
So let us finally write the same program using array notation, for those who prefer array notation more over pointer notation.
Program to input and print array using pointer in array notation
/**
* C program to input and print array elements using pointer in array notation
*/
#include <stdio.h>
#define MAX_SIZE 100 // Maximum array size
int main()
{
int arr[MAX_SIZE];
int N, i;
int * ptr = arr; // Pointer to arr[0]
printf("Enter size of array: ");
scanf("%d", &N);
printf("Enter elements in array:\n");
for (i = 0; i < N; i++)
{
// &ptr[i] is equivalent to &arr[i]
scanf("%d", &ptr[i]);
}
printf("Array elements: ");
for (i = 0; i < N; i++)
{
// i[ptr] is equivalent to arr[i], i[arr] and ptr[i]
// I have used i[ptr] syntax for knowledge. You can also use ptr[i]
printf("%d, ", i[ptr]);
}
return 0;
}
Before you move on make sure you have learned to read and print array using recursion.
Output
Enter size of array: 10 Enter elements in array: 1 2 3 4 5 6 7 8 9 10 Array elements: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10,