Write a C program to count nodes in Circular Linked List. In this example I will explain how to count nodes in Circular Linked List. C program to create a Circular Linked List and count total elements in Circular Linked List.
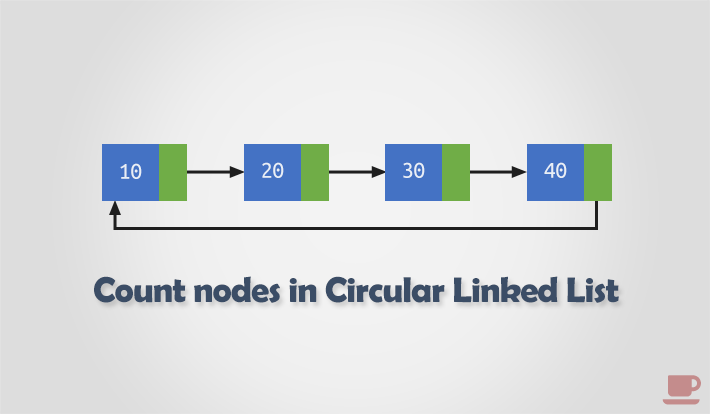
Required knowledge
Do while loop, Functions, Pointers, Structures, Dynamic Memory Allocation
Logic to count nodes in a Circular Linked List
Step by step descriptive logic to count nodes in a circular linked list.
- Create a Circular Linked List and assign reference of first node to head.
- Initialize
count = 0;
variable to store total nodes in list. - Initialize another variable to traverse list, say
current = head;
. - Increment
count++
andcurrent = current->next;
. - Repeat step 4 till you reach head node after traversing once.
Program to count nodes in Circular Linked List
/**
* C program to count nodes in a Circular Linked List
*/
#include <stdio.h>
#include <stdlib.h>
/* Basic structure of node */
struct node {
int data;
struct node * next;
};
/* Function declaration */
void createList(struct node **head, int n);
void displayList(struct node *head);
int count(struct node *head);
int main()
{
int n, choice;
struct node *head = NULL;
/*
* Run forever until user chooses 0
*/
while(choice != 0)
{
printf("--------------------------------------------\n");
printf(" CIRCULAR LINKED LIST PROGRAM \n");
printf("--------------------------------------------\n");
printf("1. Create List\n");
printf("2. Display list\n");
printf("3. Count nodes\n");
printf("0. Exit\n");
printf("--------------------------------------------\n");
printf("Enter your choice : ");
scanf("%d", &choice);
switch(choice)
{
case 1:
printf("Enter total node to create: ");
scanf("%d", &n);
createList(&head, n);
break;
case 2:
displayList(head);
getchar(); // Hold screen
getchar(); // Hold screen
break;
case 3:
printf("Total nodes = %d\n", count(head));
getchar(); // Hold screen
getchar(); // Hold screen
break;
case 0:
printf("Exiting from application");
exit(0);
break;
default:
printf("Error! Invalid choice. Please choose between 0-3");
}
printf("\n\n\n\n\n");
}
return 0;
}
/**
* Function to count total number of nodes in Circular Linked List
*/
int count(struct node *head)
{
int total = 0;
struct node *current = head;
// Iterate till end of list
do
{
current = current->next;
total++;
} while (current != head);
// Return total nodes in list
return total;
}
/**
* Creates a circular linked list of n nodes.
*/
void createList(struct node **head, int n)
{
int i, data;
struct node *prevNode, *newNode;
prevNode = NULL;
newNode = NULL;
/* Creates and links rest of the n-1 nodes */
for(i=1; i<=n; i++)
{
// Create a new node
newNode = (struct node *) malloc(sizeof(struct node));
printf("Enter data of %d node: ", i);
scanf("%d", &data);
newNode->data = data;
newNode->next = NULL;
// Link the previous node with newly created node
if (prevNode != NULL)
prevNode->next = newNode;
// Move the previous node ahead
prevNode = newNode;
// Link head node if not linked
if (*head == NULL)
*head = newNode;
}
// Link last node with first node
prevNode->next = *head;
printf("\nCIRCULAR LINKED LIST CREATED SUCCESSFULLY\n");
}
/**
* Display node content of circular linked list
*/
void displayList(struct node *head)
{
struct node *current;
int n = 1;
// Nothing to print in list
if(head == NULL)
{
printf("List is empty.\n");
return;
}
current = head;
printf("DATA IN THE LIST:\n");
do
{
// Print current node
printf("Data %d = %d\n", n++, current->data);
// Move to next node
current = current->next;
} while (current != head);
}
-------------------------------------------- CIRCULAR LINKED LIST PROGRAM -------------------------------------------- 1. Create List 2. Display list 3. Count nodes 0. Exit -------------------------------------------- Enter your choice : 1 Enter total node to create: 5 Enter data of 1 node: 10 Enter data of 2 node: 20 Enter data of 3 node: 30 Enter data of 4 node: 40 Enter data of 5 node: 50 CIRCULAR LINKED LIST CREATED SUCCESSFULLY -------------------------------------------- CIRCULAR LINKED LIST PROGRAM -------------------------------------------- 1. Create List 2. Display list 3. Count nodes 0. Exit -------------------------------------------- Enter your choice : 2 DATA IN THE LIST: Data 1 = 10 Data 2 = 20 Data 3 = 30 Data 4 = 40 Data 5 = 50 -------------------------------------------- CIRCULAR LINKED LIST PROGRAM -------------------------------------------- 1. Create List 2. Display list 3. Count nodes 0. Exit -------------------------------------------- Enter your choice : 3 Total nodes = 5 -------------------------------------------- CIRCULAR LINKED LIST PROGRAM -------------------------------------------- 1. Create List 2. Display list 3. Count nodes 0. Exit -------------------------------------------- Enter your choice : 0 Exiting from application