Write a Java program to input radius of circle and find diameter, circumference and area of circle. Logic to find diameter circumference and area of circle.
Input
Enter radius of circle : 6
Output
Diameter of circle is : 20 Circumference of circle is : 62.8318 Area of circle is : 314.159
Required knowledge
Arithmetic operators, Data types, Basic Input/Output
Components of circle
Before we write Java code, let us recall basic components of circle. And how they are mathematically related with each other.
- A circle has a radius, denoted with letter r in mathematics.
- Circle has a diameter, denoted by letter d. Relation between diameter and radius of circle is given by the equation
d = 2 * r
. - Circumference is the boundary of circle. Formulae for calculating circumference is written as
circumference = 2 * PI * r
. Here PI is constant equal to 3.141592653. - Area of circle is evaluated by equation
area = PI * r * r
.
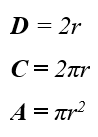
Program to find diameter circumference and area of circle
/**
* Program to find diameter, circumference and area of circle.
*/
import java.util.Scanner;
public class Circle {
public static void main(String[] args) {
// Declare constant for PI
final double PI = 3.141592653;
Scanner in = new Scanner(System.in);
/* Input radius of circle from user. */
System.out.println("Please enter radius of the circle : ");
int r = in.nextInt();
/* Calculate diameter, circumference and area. */
int d = 2 * r;
double circumference = 2 * PI * r;
double area = PI * r * r;
/* Print diameter, circumference and area of circle. */
System.out.println("Diameter of circle is : " + d);
System.out.println("Circumference of circle is : " + circumference);
System.out.println("Area of circle is : " + area);
}
}
Here we are declaring a constant variable PI through final
keyword.
Instead of declaring our custom constant for PI, we can Java predefined constant for PI, defined in Math class i.e. Math.PI
.
Math is built-in class in java, available in java.lang
package. PI is a static final double
type variable of Math. Because PI is static
, so we can use it without creating object of Math class. And PI is final
also, so we cannot change its value in our program.
Output
Please enter radius of the circle : 20 Diameter of circle is : 40 Circumference of circle is : 125.66370612 Area of circle is : 1256.6370612
Happy coding 😉