Write a C program to implement stack data structure with push and pop operation. In this post I will explain stack implementation using array in C language.
In my previous data structures examples, we learnt about Linked List (singly, doubly and circular). Here, in this post we will learn about stack implementation using array in C language.
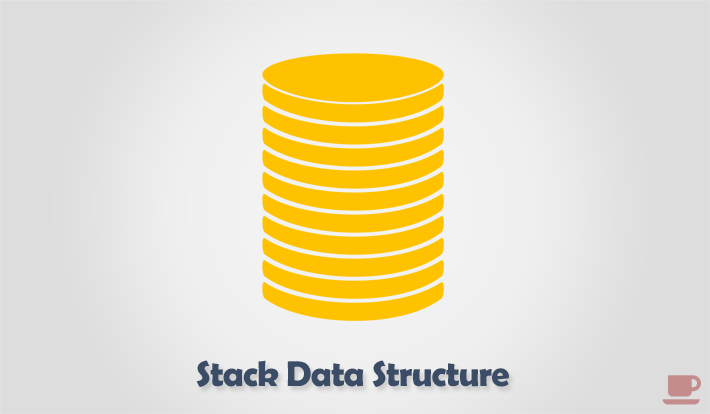
Required knowledge
Array, For loop, Functions, Stack
What is Stack?
Stack is a LIFO (Last In First Out) data structure. It allows us to insert and remove an element in special order. Stack allows element addition and removal from the top of stack.
Operations performed on Stack
In this post I will explain the stack creation, push and pop operations using array in C language. Following are the operations we generally perform on stack data structure.
How to create stack data structure using array?
Example:
int stack[SIZE];
The above code creates a stack of integer. Where SIZE is the capacity of stack.
As you know stack follows the concept of LIFO. Hence, we will need a variable to keep track of top element. Let, us declare a variable top to keep track of top element.
int top = -1;
In the above code I have initialized top with -1. Which means there are no elements in stack.
How to push elements to stack?
Inserting/Adding a new element to stack is referred as Push operation in stack. Step by step descriptive logic to push element to stack.
- If stack is out of capacity i.e.
top >= SIZE
, then throw “Stack Overflow” error. Otherwise move to below step. - Increment top by one and push new element to stack using
stack[++top] = data;
(where data is the new element).
How to pop elements from stack?
Removing an element from stack is referred as Pop operation in stack. Step by step descriptive logic to pop element from stack.
- If
top < 0
, then throw “Stack is Empty” error. Otherwise move down to below step. - Return the top most element from stack and decrement top by one. Say
return stack[top--];
.
How to find size of stack?
Unlike linked list and other data structures. Finding size of stack is straightforward. Size of stack is represented by top. Since we have initialized top = -1;, hence size of stack will be top + 1
.
Note: Element are always pushed/added on top of stack. And elements are always popped/removed from top of stack.
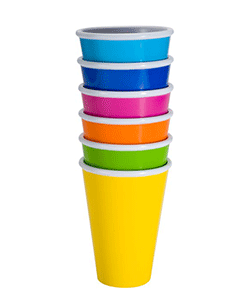
The above image represents stack of cups. You can only add or remove from the top of stack.
Stack implementation using array program in C
/**
* Stack implementation using array in C language.
*/
#include <stdio.h>
#include <stdlib.h>
#include <limits.h> // For INT_MIN
#define SIZE 100
// Create a stack with capacity of 100 elements
int stack[SIZE];
// Initially stack is empty
int top = -1;
/* Function declaration to perform push and pop on stack */
void push(int element);
int pop();
int main()
{
int choice, data;
while(1)
{
/* Menu */
printf("------------------------------------\n");
printf(" STACK IMPLEMENTATION PROGRAM \n");
printf("------------------------------------\n");
printf("1. Push\n");
printf("2. Pop\n");
printf("3. Size\n");
printf("4. Exit\n");
printf("------------------------------------\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice)
{
case 1:
printf("Enter data to push into stack: ");
scanf("%d", &data);
// Push element to stack
push(data);
break;
case 2:
data = pop();
// If stack is not empty
if (data != INT_MIN)
printf("Data => %d\n", data);
break;
case 3:
printf("Stack size: %d\n", top + 1);
break;
case 4:
printf("Exiting from app.\n");
exit(0);
break;
default:
printf("Invalid choice, please try again.\n");
}
printf("\n\n");
}
return 0;
}
/**
* Functiont to push a new element in stack.
*/
void push(int element)
{
// Check stack overflow
if (top >= SIZE)
{
printf("Stack Overflow, can't add more element element to stack.\n");
return;
}
// Increase element count in stack
top++;
// Push element in stack
stack[top] = element;
printf("Data pushed to stack.\n");
}
/**
* Function to pop element from top of stack.
*/
int pop()
{
// Check stack underflow
if (top < 0)
{
printf("Stack is empty.\n");
// Throw empty stack error/exception
// Since C does not have concept of exception
// Hence return minimum integer value as error value
// Later in code check if return value is INT_MIN, then
// stack is empty
return INT_MIN;
}
// Return stack top and decrease element count in stack
return stack[top--];
}
------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 2 Stack is empty. ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 1 Enter data to push into stack: 10 Data pushed to stack. ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 1 Enter data to push into stack: 20 Data pushed to stack. ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 1 Enter data to push into stack: 30 Data pushed to stack. ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 3 Stack size: 3 ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 2 Data => 30 ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 2 Data => 20 ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 2 Data => 10 ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 2 Stack is empty. ------------------------------------ STACK IMPLEMENTATION PROGRAM ------------------------------------ 1. Push 2. Pop 3. Size 4. Exit ------------------------------------ Enter your choice: 4 Exiting from app.