Write a C program to swap two numbers using macro. How swap two numbers without using third variable using macro in C program. Logic to swap two number without using third variable using macro.
Swapping values of two variables is a common problem. We already discussed several ways to swap two variables throughout the course of C programming tutorial.
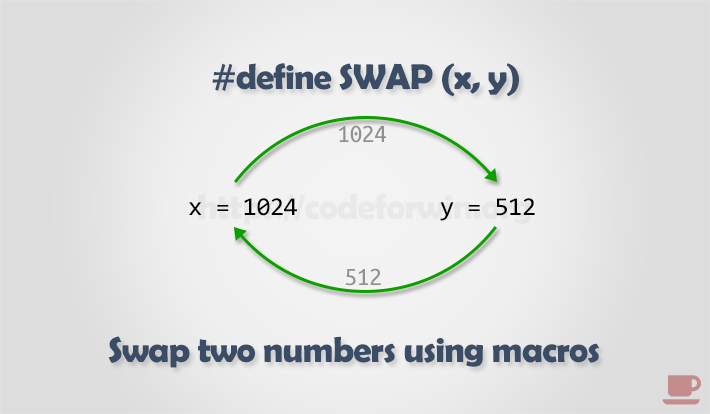
Learn different ways to swap
In this post we will continue our macro exercise. Here I will explain how you can transform swapping logic to macro.
Required knowledge
Basic C programming, Macros, Bitwise operator
How to swap two numbers using macro
Before moving ahead, I assume that you are aware with macro syntax, how to define and use.
For this post I will swap two numbers without using third variable. I will make use of bitwise operator. If you have any related to bitwise operator logic please read how to swap two numbers using bitwise operator.
Let us get started and define a macro that accepts two arguments say SWAP(x, y)
. The macro will swap the values of x
and y
.
Example:
#define SWAP(x, y) (x ^= y ^= x)
Program to swap two numbers using macro
/**
* C program to swap two numbers using macro
*/
#include <stdio.h>
// Define macro to swap two numbers
#define SWAP(x, y) (x ^= y ^= x ^= y)
int main()
{
int num1, num2;
// Input two numbers from users
printf("Enter any two number to swap: ");
scanf("%d%d", &num1, &num2);
printf("Values before swapping\n");
printf("num1 = %d, num2 = %d\n\n", num1, num2);
SWAP(num1, num2);
printf("Values after swapping\n");
printf("num1 = %d, num2 = %d\n", num1, num2);
return 0;
}
Read more
Output
Enter any two number to swap: 10 20 Values before swapping num1 = 10, num2 = 20 Values after swapping num1 = 20, num2 = 10
Happy coding 😉