Quick links
Data type is a system for defining various basic properties about the data stored in memory. Properties such as, type of data, range of data, bytes occupied, how these bytes are interpreted etc.
For example: int
is a data type used to define integer type variables.
int a;
here a is an integer type variable. It can store numbers from -2,147,483,648 to +2,147,483,647.
Data types in C is classified in three broad categories.
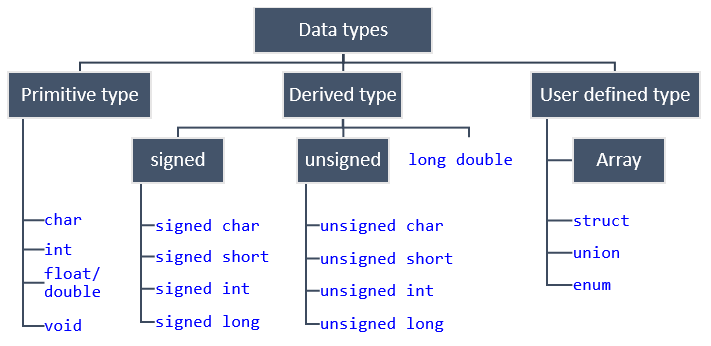
Read more – List of all primitive and derived data types in C.
Primitive data type
C language supports four primitive types – char
, int
, float
, void
. Primitive types are also known as pre-defined or basic data types.
Data type | Size | Range | Description |
---|---|---|---|
char | 1 byte | -128 to +127 | A character |
int | 2 or 4 byte | -32,768 to 32,767 or -2,147,483,648 to +2,147,483,647 | An integer |
float | 4 byte | 1.2E-38 to 3.4E+38 | Single precision floating point number |
void | 1 byte | void type stores nothing |
The size and range of a data type is machine dependent and may vary from compiler to compiler. C standard requires only the minimum size to be fulfilled by every compiler for each data type. For example, size of int
type varies from compiler to compiler, but it must be at least 2 bytes on every compiler.
Character type char
Any single character value in C is represented using char
. Size of char
type is 1 byte and can store 128 characters.
Example to define char
type variable –
char grade = 'A';
In above code grade
is defined as a character type variable and can store any character.
Read more – How to declare character literal (value)?
Integer type int
In C programming int
keyword is used to define a number type. Size of int
is 2 or 4 bytes (compiler dependent) and can store values up to -32,768 to 32,767 or -2,147,483,648 to +2,147,483,647.
Example to define int
type variable –
int roll = 24;
In above code roll
is defined as an integer type variable and can store any number in int
range.
Float type float
A real type value in C is defined with float
or double
keyword. float
defines a single precision floating point number that can store 1.2E-38 to 3.4E+38. Whereas double
defines a double precision floating point number that can store 2.3E-308 to 1.7E+308.
Number of significant digits after decimal point is known as its precision. Precision of float
type is of 6 decimal places and double
is of 15 decimal places.
Example to define float
type variable –
float percentage = 95.67;
const float PI = 3.14159265359f;
double speed = 2.998e+8;
C considers floating point literal as
double
type. Add suffixf
orF
after floating point literal to specify type asfloat
.
void
type
As name suggests void
internally does not store anything. void
keyword is used to define a function return type or a generic pointer.
Example to define void
pointer type –
void * ptr;
In the above code ptr
is defined as a void
pointer. We will learn about void
types in later section of this programming tutorial series.
Derived data types
A derived data type is defined using combination of qualifiers along with the primitive data type. Derived types are created using basic data types with modified behaviour and property.
Data type Qualifiers
Qualifiers are optional add-on to the basic data types. They are used to alter the behaviour and property of basic data types yielding new type with new property and behaviour.
There are two types of data type qualifiers in C, size and sign qualifier. They are used along with the basic data types in any of the two given syntax.
[qualifier] <basic-data-type>
OR
<basic-data-type> [qualifier]
Standard syntax to use a qualifier
[sign-qualifier] [size-qualifier] <basic-data-type>
Example: unsigned short int
Note: Parts in the square brackets [
]
are optional and parts in angle bracket <
>
are mandatory.
Size qualifier
Size qualifier in C is used to alter size of a primitive data type. C supports two size qualifier, short
and long
.
Size qualifier is generally used with integer type. In addition, double
type supports long
qualifier.
Syntax to use size qualifier
[size-qualifier] <basic-data-type>
OR
<basic-data-type> [size-qualifier]
Example to use size qualifier
Data type | Description |
---|---|
short int | Defines min 2 bytes integer |
long int | Defines a min 4 bytes integer |
long double | Defines a min 12 bytes double precision floating point number |
Rules regarding size qualifier as per ANSI C standard
- Size of
short
integer typeshort int
is at least 2 bytes and must be less than or equal to the size ofint
- The size of integer type
int
is at least 2 bytes and must be greater than or equal to the size ofshort
. - The size of
long
integer typeslong int
is at least 4 bytes and must be greater than or equal to the size ofint
. - The precision of
long double
must be greater than or equal todouble
. Precision ofdouble
must be greater or equal to the precision offloat
.
Note: short int
may also abbreviated as short
and long int
as long
. But, there is no abbreviation for long double
.
The GCC C compiler supports one more derived integer type long long
or long long int
. Size of long long
is 8 bytes and can store numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Must read –
Sign qualifier
Sign qualifier in C is used to specify signed nature of integer types. It specifies whether a variable can hold negative value or not.
Sign qualifiers are used with integer int
and character char
type.
C supports two sign qualifier, signed
and unsigned
. signed
specifies a variable can hold both positive as well as negative integers. unsigned
specifies a variable will only hold positive integers.
Example to use sign qualifier
signed short int
unsigned long
unsigned char
By default, integer and character types are signed in nature. Hence, using signed
keyword is useless. However, a good programmer will use to explicitly specify signed nature of the variable.
User defined data type
Despite of several basic and derived type, C language supports feature to define our custom type based on our need. User defined type include array, pointer, structures, unions, enumeration types etc.
At this point with little knowledge of C programming, it is impossible to explain user defined types. I will cover them all in upcoming sections of this tutorial series.